Deploy faster
Everything you need to deploy your app
Mobile friendly
Responsive designs that look great and work smoothly on all devices—phones, tablets, and desktops.
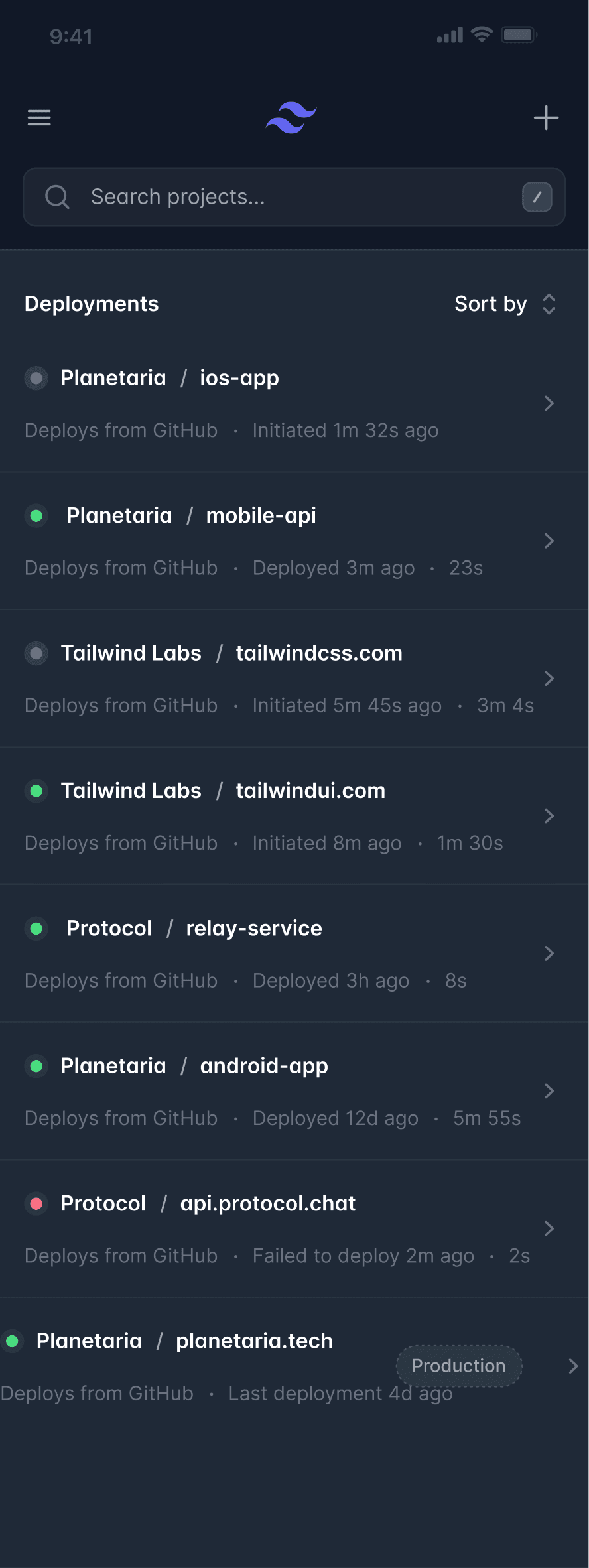
Performance & SEO
Optimized code for fast loading speeds and better search engine visibility to help users find you faster.
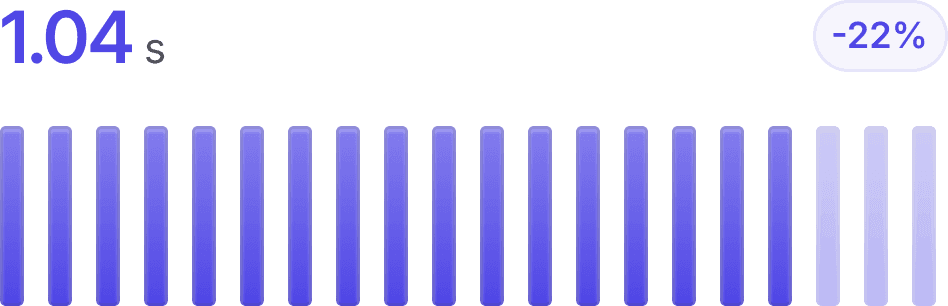
Security
Best practices and modern tools to protect your site and user data from common vulnerabilities.
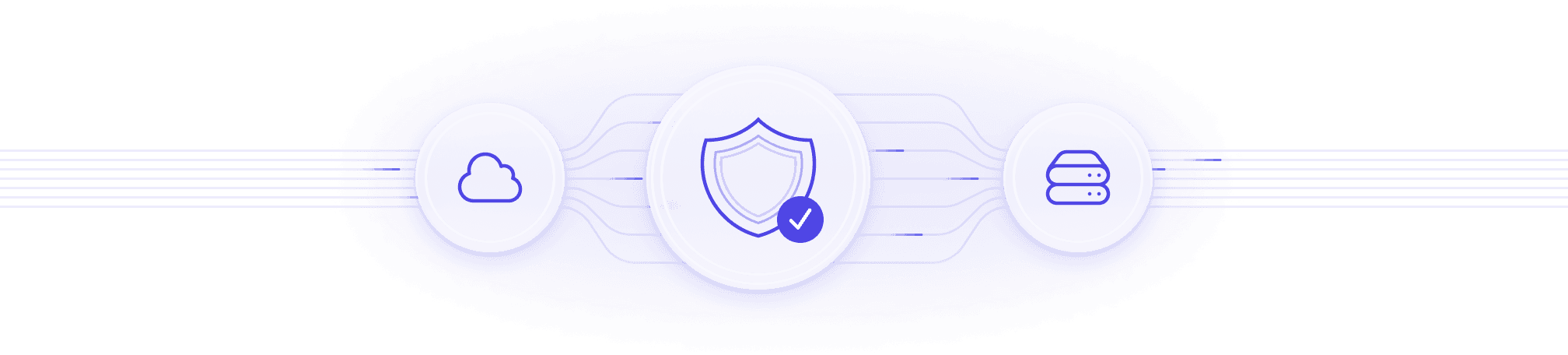
Powerful APIs
Seamless integration with third-party services and custom APIs to power dynamic, scalable features.
NotificationSetting.ts
App.ts
import express, { Request, Response } from 'express';
import mongoose from 'mongoose';
const router = express.Router();
const userSettingsSchema = new mongoose.Schema({
userId: { type: String, required: true, unique: true },
notificationsEnabled: { type: Boolean, default: false },
});
const UserSettings = mongoose.model('UserSettings', userSettingsSchema);
router.put('/notification-settings', async (req: Request, res: Response) => {
try {
const { userId, notificationsEnabled } = req.body;
if (typeof notificationsEnabled !== 'boolean') {
return res.status(400).json({ error: 'notificationsEnabled must be a boolean' });
}
const updatedSettings = await UserSettings.findOneAndUpdate(
{ userId },
{ notificationsEnabled },
{ new: true, upsert: true } // Create if not exists
);
res.status(200).json(updatedSettings);
} catch (err) {
res.status(500).json({ error: 'Server error', details: err });
}
});
export default router;